Introduction
This article deals with creation of Spark and PDF Extent Report for REST Assured validation by using a Maven Plugin. The artifact uses a custom filter based on the Allure Framework REST Assured Filter for generating the request and response data. This works with JUnit 4, JUnit 5 and TestNG testing frameworks.
This allows the test code to avoid mixing with Extent calls. All that is required in the code is an addition of a REST Assured filter. The remaining changes are all POM configuration changes.
In addition to the usual test statistics, request and response data is also displayed. These includes body, headers, parameters, cookies and multi-part information.
The article is divided into four sections – Report structure summary, POM configuration, Report generation, Additional report details.
To generate Spark and PDF reports with Cucumber-JVM with Rest Assured execution refer to this article.
Source Code
The source code for the report Maven plugin is located here. The source code for the sample implementations for JUnit 4, JUnit 5 and TestNG. The sample reports for JUnit 4, Junit 5 and TestNG.
In case you are upgrading from version 1.6.0 or lower, you will need to change one dependency in the POM and change the name of the filter. From version 2.0.0 onwards, dependency ‘extent-allure-rest-assured‘ with groupid ‘tech.grasshopper’ will replace the existing ‘allure-rest-assured’ dependency and in the test code, change ‘AllureRestAssuredFilter’ with ‘ExtentRestAssuredFilter‘.
Report Structure Summary
The Spark and Pdf report both use the CLASS Analysis Strategy which broadly means that only two levels of tests are used. In this case the primary level is the test class and the secondary level is the test method. The reports do not take test suites into account.
The report supports the categories, authors and devices view with the help of Allure label custom annotations. The system information or environment data can be displayed with the help of a properties file.
Both the reports are enabled by default. This can be changed in the maven plugin configuration.
Spark Report
The Spark report is enabled by default and this can be disabled with the ‘generateSpark‘ plugin configuration. The ‘sparkConfigFilePath‘ can be used for the report configuration. More details can be found in ‘Maven Report Plugin Configurations’ section below. These are the sample of the Spark report for JUnit 4, JUnit 5 and Test NG.
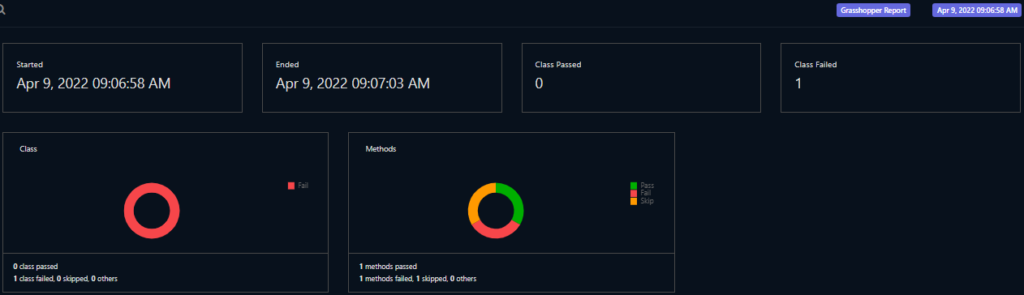
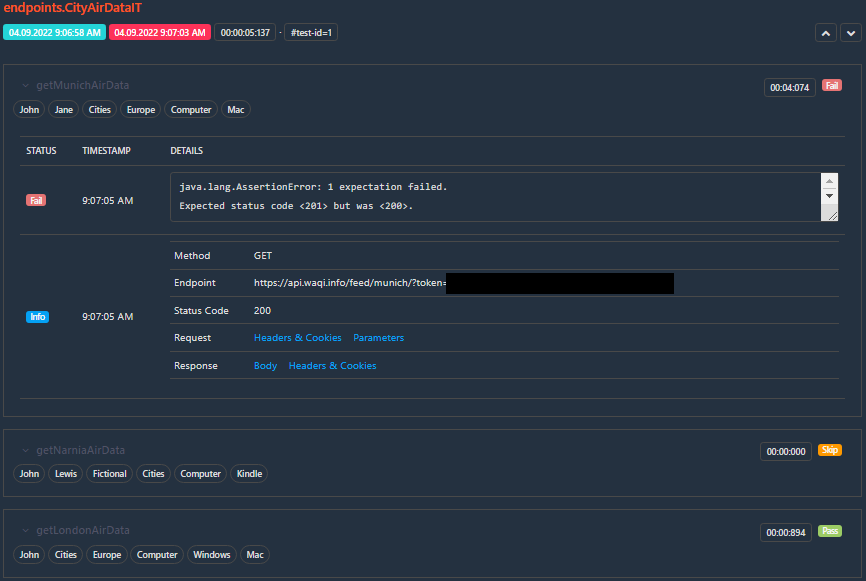
PDF Report
The Pdf report is enabled by default and this can be disabled with the ‘generatePdf‘ plugin configuration. The ‘pdfConfigFilePath‘ can be used for the report configuration. More details can be found in ‘Maven Report Plugin Configurations’ section below. These are the sample of the PDF report for JUnit 4, JUnit 5 and Test NG.
The configuration options for the report can be found in this article, refer to the ‘Configurations’ section.
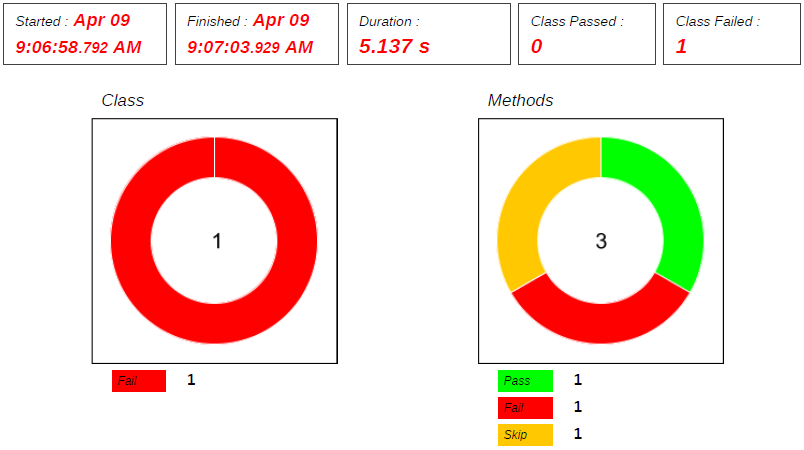

POM Configuration
It is assumed that the REST Assured dependency and the relevant testing framework (JUnit4, JUnit5 or TestNG) has already been added. Following are the steps for configuring the test project POM.
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>rest-assured</artifactId>
<version>4.4.0</version>
</dependency>
1. Dependency
The only dependency that needs to be added to the test project, from version 2.1.2 onwards, is the rest-assured-extent-junit4 for JUnit 4, rest-assured-extent-junit5 for JUnit5 and rest-assured-extent-testng for TestNG.
For previous versions, the supporting jars need to be added individually. Refer to the sample POM for more details – JUnit4, JUnit5 & TestNG.
- extent-allure-rest-assured – This artifact contains the custom Rest Assured filter. This dependency is required from version 2.0.0 onwards, which replaced the ‘allure-rest-assured‘ artifact used in 1.x.x versions.
- allure listener – Use allure-junit4 for JUnit4, allure-junit5 for JUnit5 and allure-testng for TestNG.
- extentreport-allure-annotation – This contains annotations for adding author, category and device information.
<dependency>
<groupId>tech.grasshopper</groupId>
<artifactId>rest-assured-extent-junit4</artifactId>
<version>1.0.0</version>
</dependency>
<dependency>
<groupId>tech.grasshopper</groupId>
<artifactId>rest-assured-extent-junit5</artifactId>
<version>1.0.0</version>
</dependency>
<dependency>
<groupId>tech.grasshopper</groupId>
<artifactId>rest-assured-extent-testng</artifactId>
<version>1.0.0</version>
</dependency>
2. Maven Failsafe Plugin Configurations
JUnit4 – Sample configuration can be found here.
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-failsafe-plugin</artifactId>
<version>3.0.0-M5</version>
<executions>
<execution>
<goals>
<goal>integration-test</goal>
<goal>verify</goal>
</goals>
</execution>
</executions>
<configuration>
<systemPropertyVariables>
<allure.results.directory>${project.build.directory}/allure-results
</allure.results.directory>
</systemPropertyVariables>
<properties>
<property>
<name>listener</name>
<value>io.qameta.allure.junit4.AllureJunit4</value>
</property>
</properties>
</configuration>
</plugin>
JUnit 5 – The configuration is similar to that for JUnit4. The listener property needs to be changed for JUnit 5. Sample configuration can be found here.
<properties>
<property>
<name>listener</name>
<value>io.qameta.allure.junit5.AllureJunit5</value>
</property>
</properties>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter</artifactId>
<version>5.8.1</version>
</dependency>
TestNG – The configuration is similar to that for JUnit 4. There is no need for the listener property which needs to be removed. Sample configuration can be found here.
3. Maven Report Plugin Configurations
<plugin>
<groupId>tech.grasshopper</groupId>
<artifactId>rest-assured-extent-report-plugin</artifactId>
<version>2.1.2</version>
<executions>
<execution>
<id>report</id>
<phase>post-integration-test</phase>
<goals>
<goal>extentreport</goal>
</goals>
</execution>
</executions>
</plugin>
An example of a POM with the below plugin configuration settings can be found here.
Parameter | Description | Default Value | Remarks |
allureResultsDirectory | Allure results directory path | target/allure-results | same value should also be in the ‘ systemPropertyVariables’ of the firesafe plugin. |
reportDirectory | Reports directory prefix | report | |
reportDirectoryTimeStamp | Reports directory timestamp pattern suffix | dd MM yyyy HH mm ss | pattern should not include ‘invalid’ file naming characters like ‘:’ etc. |
systemInfoFilePath | Reports system info properties | src/test/resources/systeminfo.properties | |
sparkGenerate | Spark report generation flag | true | |
sparkConfigFilePath | Spark report configuration file | src/test/resources/spark-config.xml | |
sparkViewOrder | Spark report view order | spark report default order | comma delimited string of values from view name. |
sparkHidelogEvents | Spark report dashboard view hide log events pie chart | true | |
pdfGenerate | Pdf report generation flag | true | |
pdfConfigFilePath | Pdf report configuration file | src/test/resources/pdf-config.xml | |
requestHeadersBlacklist | Hide request headers value | comma delimited string of request header names to blacklist | |
responseHeadersBlacklist | Hide response headers value | comma delimited string of response header names to blacklist |
Report Generation
In this article, we will be using the publically available REST API for Air Quality data. To access this API, a token is required which can be generated from this page by supplying a valid email id. This token needs to be passed as a query parameter. Feel free to use any other REST API available to you.
1. Extent Allure REST Assured Filter
Below is a snippet from the test class located here. The ExtentRestAssured filter captures the request and response to store them in the allure results directory.
@Categories({ @Category("Cities") })
@Authors({ @Author("John") })
@Devices({ @Device("Computer") })
public class CityAirDataIT {
@Test
@Categories({ @Category("Europe") })
@Devices({ @Device("Mac"), @Device("Windows") })
public void getLondonAirData() {
given().param("token", <token>).filter(new ExtentRestAssuredFilter()).cookie("Cookie 1", "cookie one")
.cookie("Cookie 2", "cookie two").header("Header 1", "header 1").when()
.get("https://api.waqi.info/feed/london/").then().statusCode(equalTo(200));
}
}
2. Test Execution
The tests can be executed with the ‘mvn clean install‘ command. The reports will be generated in the designated directory (reportDirectory reportDirectoryTimestamp).
It is advisable to place the allureResultsDirectory inside the project build directory so it can be deleted with the ‘clean‘ command. If the contents of the folder are not deleted then previous execution results will be displayed in the report.
3. Sample Report
Below is a part of the report generated from the execution of the above class. The request and response body contents are displayed as a browser pop up. The headers, cookies, parameters are displayed similarly as pop ups too, in a tabular fashion. These are available as links in the Spark report. In the Pdf report, the details are available by clicking on the pin annotations. The details are shown in a browser pop up. The files are embedded inside the Pdf report and can also be viewed from View -> Navigation Panels -> Attachments.
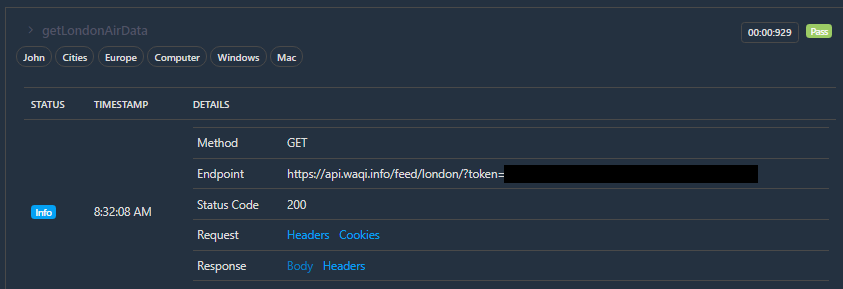
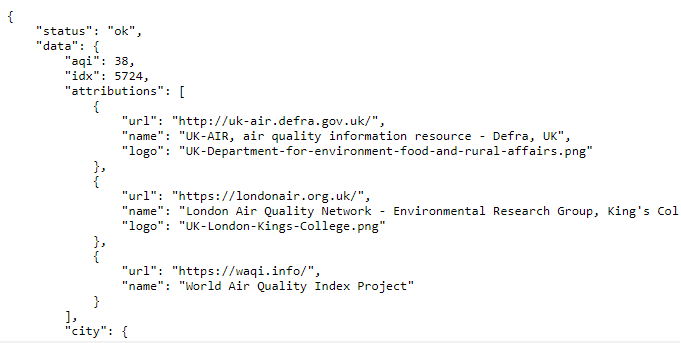
Report Additional Details
Following are some points which can be helpful in successful report generation.
1. Allure Results Directory
This is set by using the allureResultsDirectory configuration in the report plugin. The default value is ‘target/allure-results‘ directory path. This value also needs to be set as a ‘systemPropertyVariables‘ in the failsafe plugin. There are two points to consider.
First, if changing the value, remember to do it in both places, or better create a property and use it in both the locations.
Second, the contents of this folder need to be deleted before the test execution. Else the report will display results from previous runs. It is advisable to place the results directory in the project build so it gets deleted with the ‘clean‘ command.
2. Configuration File
The default path for the Spark report configuration is ‘src/test/resources/spark-config.xml‘. Place a file with the same name and location, will be picked up automatically. A custom file name and location can be set by using the ‘sparkConfigFilePath‘ configuration. If using a JSON file, this will need to be added.
Similarly for Pdf report the configuration is ‘pdfConfigFilePath‘ and the default value is ‘ src/test/resources/pdf-config.xml ‘.
3. System Info Properties File
The default path for this configuration is ‘src/test/resources/systeminfo.properties‘. Placing a file with the same name and location will be picked up automatically. A custom file name and location can be set by using the ‘systemInfoFilePath‘ configuration.
4. Category, Author, Device Annotation
The category, author and device views are automatically displayed by using these custom annotations. These annotations are based on the Label Allure annotation.
5. Blacklist Request and Response Headers
The header values can be hidden by using the ‘requestHeadersBlacklist’ and ‘responseHeadersBlacklist’ configuration. Multiple values can be added by using a comma delimited string as value. The value will be hidden by using the [BLACKLIST] text.
6. Spark Report View Order
The view order can be changed by using the ‘sparkViewOrder‘ configuration. The comma delimited values are from the ViewName enum. The default order is ‘TEST, CATEGORY, AUTHOR, DEVICE, EXCEPTION, DASHBOARD‘.
7. JUnit 5 Nested Tests
The tests inside a nested test class will be treated as a separate class. The nested tests will not be included in the containing class.
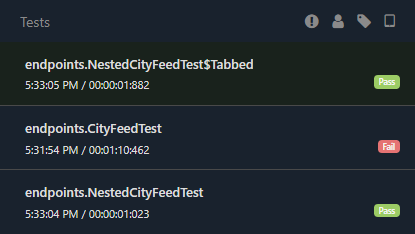
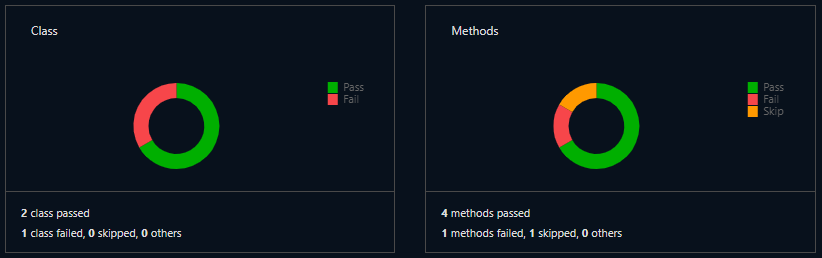
Hi,
is it possible using rest-assured-extent-report-plugin, also to attach image screenshoot for combined test (api and mobile)?
Because I’ve tried but it seems that the plugin doesn’t process other attachment type.
Is There a way to combine the two test?
Thanks.
Spark & Pdf Extent Report generation for REST Assured API Testing executed with Cucumber-JVM
Is thr any sample/example project for the cucumber JVM restassuured Plugin?
https://github.com/grasshopper7/cucumber-rest-assured-extent-report-plugin
Getting stuff ready. Meanwhile you can have a look at these. https://github.com/grasshopper7/cucumber-rest-assured-testng-report OR https://github.com/grasshopper7/cucumber-rest-assured-junit-report. It has a sample report, download and have a look. Documentation should be done in 3-4 days.
Check this – https://ghchirp.site/4199/
This is my pom file. i am not able to get this report.
4.0.0
org.example
PropertyV2
1.0-SNAPSHOT
1.8.10
1.8
1.8
${project.build.directory}\allure-results
${project.build.directory}\extentreport
io.rest-assured
json-schema-validator
4.3.1
tech.grasshopper
extentreport-allure-annotation
1.0
commons-io
commons-io
2.11.0
com.fasterxml.jackson.dataformat
jackson-dataformat-xml
2.13.1
org.skyscreamer
jsonassert
1.5.0
test
org.wiztools
xsd-gen
0.2.1
org.apache.poi
poi-ooxml
5.0.0
org.testng
testng
7.4.0
org.slf4j
slf4j-simple
1.7.32
org.apache.commons
commons-lang3
3.12.0
com.aventstack
extentreports
5.0.9
org.json
json
20210307
com.google.code.gson
gson
2.8.8
com.github.saasquatch
json-schema-inferrer
0.1.4
commons-validator
commons-validator
1.7
org.bitbucket.cowwoc
diff-match-patch
1.2
io.qameta.allure
allure-rest-assured
2.17.2
io.qameta.allure
allure-testng
2.17.2
io.rest-assured
rest-assured
4.4.0
compile
org.apache.maven.plugins
maven-surefire-plugin
3.0.0-M5
qatestng.xml
true
org.apache.maven.plugins
maven-compiler-plugin
1.8
1.8
org.apache.maven.plugins
maven-failsafe-plugin
3.0.0-M5
integration-test
verify
target/test
tech.grasshopper
rest-assured-extent-report-plugin
1.4.0
report
post-integration-test
extentreport
target/test
target/extent
dd MM yyyy HH mm ss
true
false
src/test/resources/spark-config.xml
src/test/resources/pdf-config.xml
src/test/resources/systeminfo.properties
DASHBOARD,TEST,EXCEPTION,CATEGORY,AUTHOR,DEVICE
true
jitpack.io
https://jitpack.io
GroupDocsJavaAPI
GroupDocs Java API
http://repository.groupdocs.com/repo/
Seems u r using testng. Have a look at the sample implementation – https://github.com/grasshopper7/rest-assured-report-testng
Hi Sir. I made all changes mentioned here but the reports are not getting generated. can you pls any link to working project
I am not able to convert this implementation with cucumber BDD + RestAssured +Spark & Pdf Extent Report generation for REST Assured.
Do you have any examples for RestAssured + cucumber BDD and your plugin for API testing?
Please extend this example with cucumber BDD and API testing framework design.
It will be a Great Help. Looking forward to your response
Plugin purpose is to generate reports for vanilla Rest Assured execution for which I could not find any simple reporting solution. I have not tried it with cucumber and I do not think this solution will work.
I have never tried Rest Assured with Cucumber. Is this basically how you are using it -> https://devqa.io/cucumber-layer-rest-assured-api-tests/. Or do u have a better example.
is there any plan for PDF report implementation to rest assured?
Yeah there is. But information will be limited. Most probably body, headers, cookies data will not be present.
The Pdf version of the report is now implemented in version 1.4.0. Details of body, headers and cookies are available as embedded files and viewable as browser pop-ups.
extentreport-allure-annotation dependency in the pom 1.0.0 to 1.0.
Much awaited problem going to be resolved, when you want selenium, cucumber, and Rest-assured with comment reporting that suits all. I’ll try it out for sure!!
Do try it out and send me your feedback.