Introduction
This article deals with creation of Spark and PDF Extent Report for REST Assured validation with Cucumber by using a Maven Plugin. The artifact uses a custom filter based on the Allure Framework REST Assured Filter for generating the request and response data. The Cucumber related data is retrieved from the Cucumber Json report.
This allows the test code to avoid mixing with Extent calls. All that is required in the code is an addition of a REST Assured filter and two Cucumber plugins. The remaining changes are all related to POM configurations.
In addition to the usual test statistics, request and response data is also displayed. These includes body, headers, parameters, cookies and multi-part information.
The article is divided into five sections – Report structure summary, POM configuration, Cucumber Runner configuration, Report generation, Additional report details.
To generate Spark and PDF reports with vanilla Rest Assured execution refer to this article.
Source Code
The source code for the report Maven plugin is located here. The source code for the sample implementations for is located here (JUnit & TestNG).
In case you are upgrading from version 1.1.1 or lower, you will need to change one dependency in the POM and change the name of the filter. From version 2.0.0 onwards, dependency ‘extent-allure-rest-assured‘ with groupid ‘tech.grasshopper’ will replace the existing ‘allure-rest-assured’ dependency and in the test code, change ‘AllureRestAssuredFilter’ with ‘ExtentRestAssuredFilter‘.
Report Structure Summary
The generated report contain Rest Assured data in addition to the Cucumber data.
The report supports the categories view with the help of Cucumber tags in the feature files. The system information or environment data can be displayed with the help of a properties file.
Both the reports are enabled by default. This can be changed in the maven plugin configuration.
Spark Report
The Spark report is enabled by default and this can be disabled with the ‘generateSpark‘ plugin configuration. The ‘sparkConfigFilePath‘ can be used for the report configuration. More details can be found in ‘Maven Report Plugin Configurations’ section below. These are the sample of the Spark report for JUnit and Test NG.
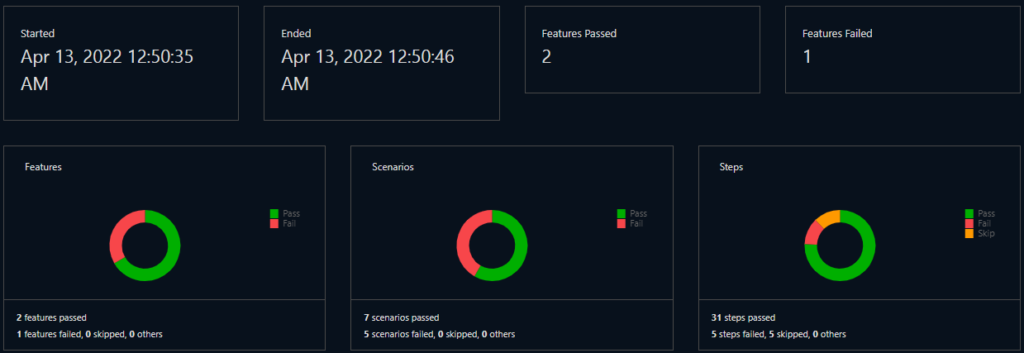
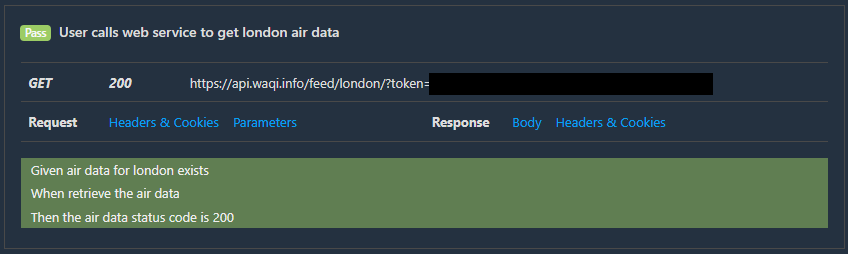
PDF Report
The Pdf report is enabled by default and this can be disabled with the ‘generatePdf‘ plugin configuration. The ‘pdfConfigFilePath‘ can be used for the report configuration. More details can be found in ‘Maven Report Plugin Configurations’ section below. These are the sample of the PDF report for JUnit and Test NG.
The configuration options for the report can be found in this article, refer to the ‘Configurations’ section.
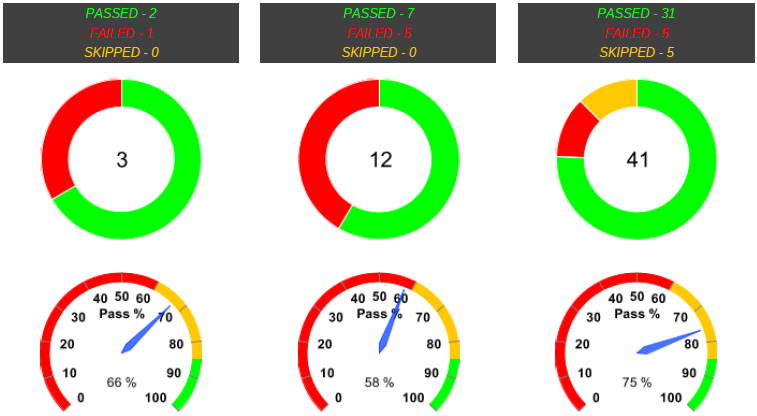
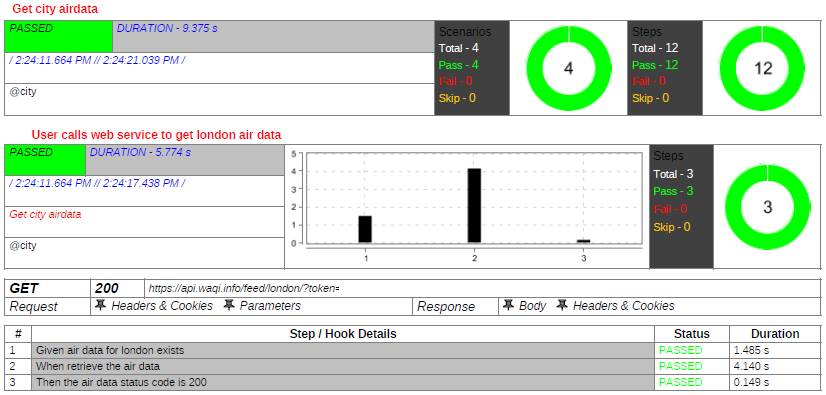
POM Configuration
It is assumed that REST Assured dependency and Cucumber-JVM dependency (JUnit or TestNG) has already been added. Following are the steps for configuring the test project POM.
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>rest-assured</artifactId>
<version>4.4.0</version>
</dependency>
1. Dependency
The only dependency that needs to be added to the test project, from version 2.2.0 onwards, is the cucumber-rest-assured-extent-junit for JUnit 4 and cucumber-rest-assured-extent-testng for TestNG.
For previous versions, the supporting jars need to be added individually. Refer to the sample POM for more details – JUnit4 & TestNG.
- extent-allure-rest-assured – This artifact contains the custom Rest Assured filter. This dependency is required from version 2.0.0 onwards, which replaced the ‘allure-rest-assured‘ artifact used in 1.x.x versions.
- allure listener – Use allure-junit4 for JUnit4 and allure-testng for TestNG.
- allure-cucumber-mapping-plugin – The dependency is a custom Cucumber plugin which maps the Cucumber scenario to the Allure test case. The details are recorded in a json file.
<dependency>
<groupId>tech.grasshopper</groupId>
<artifactId>cucumber-rest-assured-extent-junit</artifactId>
<version>1.0.0</version>
</dependency>
<dependency>
<groupId>tech.grasshopper</groupId>
<artifactId>cucumber-rest-assured-extent-testng</artifactId>
<version>1.0.0</version>
</dependency>
2. Maven Failsafe Plugin Configurations
JUnit – Sample configuration can be found here.
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-failsafe-plugin</artifactId>
<version>3.0.0-M5</version>
<executions>
<execution>
<goals>
<goal>integration-test</goal>
<goal>verify</goal>
</goals>
</execution>
</executions>
<configuration>
<systemPropertyVariables>
<allure.results.directory>${project.build.directory}/allure-results
</allure.results.directory>
</systemPropertyVariables>
<properties>
<property>
<name>listener</name>
<value>io.qameta.allure.junit4.AllureJunit4</value>
</property>
</properties>
</configuration>
</plugin>
TestNG – The configuration is similar to that for JUnit. There is no need for the listener property which needs to be removed. Sample configuration can be found here.
3. Maven Report Plugin Configurations
<plugin>
<groupId>tech.grasshopper</groupId>
<artifactId>cucumber-rest-assured-extent-report-plugin</artifactId>
<version>2.3.1/version>
<executions>
<execution>
<id>report</id>
<phase>post-integration-test</phase>
<goals>
<goal>extentreport</goal>
</goals>
</execution>
</executions>
</plugin>
An example of a POM with the below plugin configuration settings can be found here.
Parameter | Description | Default Value | Remarks |
cucumberReportsDirectory | Cucumber JSON report directory | target | same value as provided in the json plugin on the runner options. |
allureResultsDirectory | Allure results directory path | target/allure-results | same value should also be in the ‘systemPropertyVariables’ of the failsafe plugin. |
cucumberAllureMappingFiles | Cucumber Allure Mapping file location and name. | target/cucumber-allure.json | same value as provided in the custom AllureCucumberMapping plugin on the runner options. For multiple runners, the value is comma separated mapping file paths. |
reportDirectory | Reports directory prefix | report | |
reportDirectoryTimeStamp | Reports directory timestamp pattern suffix | dd MM yyyy HH mm ss | pattern should not include ‘invalid’ file naming characters like ‘:’ etc. |
systemInfoFilePath | Reports system info properties | src/test/resources/systeminfo.properties | |
sparkGenerate | Spark report generation flag | true | |
sparkConfigFilePath | Spark report configuration file | src/test/resources/spark-config.xml | |
sparkViewOrder | Spark report view order | spark report default order | comma delimited string of values from view name. |
pdfGenerate | Pdf report generation flag | true | |
requestHeadersBlacklist | Hide request headers value | comma delimited string of request header names to blacklist | |
responseHeadersBlacklist | Hide response headers value | comma delimited string of response header names to blacklist |
Cucumber Runner Configuration
The report requires the Cucumber Json plugin and the Allure Cucumber Mapping plugin to be added to the CucumberOptions annotation on the runner class. This setup for a single runner will work with the default configuration values of the plugin.
@CucumberOptions(plugin = { "json:target/cucumber-json.json", "tech.grasshopper.AllureCucumberMappingPlugin:target/cucumber-allure.json" })
In case of a single runner with different values for the runner plugins, these will need to be configured in the maven plugin.
@CucumberOptions(plugin = { "json:cuke-json/cucumber-json.json", "tech.grasshopper.AllureCucumberMappingPlugin:target/cucumber-allure-mapping.json" })
<configuration>
<cucumberReportsDirectory>cuke-json</cucumberReportsDirectory>
<cucumberAllureMappingFiles>target/cucumber-allure-mapping.json</cucumberAllureMappingFiles>
</configuration>
In case of multiple cucumber runners, the names of the cucumber-json report and cucumber-allure-mapping files must all be different. Else the Extent reports will not display the complete results. The cucumber-allure-mapping files need to be configured as a comma separated string for the configuration cucumberAllureMappingFiles.
Support for multiple runners was added in version 2.3.0. Please note the ‘s’ at the end of the configuration parameter. The old parameter cucumberAllureMappingFile has been removed.
@CucumberOptions(plugin = { "json:cuke-json/cucumber-json1.json", "tech.grasshopper.AllureCucumberMappingPlugin:target/cucumber-allure-mapping1.json" })
@CucumberOptions(plugin = { "json:cuke-json/cucumber-json2.json", "tech.grasshopper.AllureCucumberMappingPlugin:target/cucumber-allure-mapping2.json" })
<configuration>
<cucumberReportsDirectory>cuke-json</cucumberReportsDirectory>
<cucumberAllureMappingFiles>target/cucumber-allure-mapping1.json,target/cucumber-allure-mapping2.json</cucumberAllureMappingFiles>
</configuration>
Report Generation
In this article, we will be using the publically available REST API for Air Quality data. To access this API, a token is required which can be generated from this page by supplying a valid email id. This token needs to be passed as a query parameter. Feel free to use any other REST API available to you.
1. Extent Allure REST Assured Filter
Below is a snippet from the step definition class located here. The AllureRestAssured filter captures the request and response to store them in the allure results directory.
private Response response;
private RequestSpecification request;
@Given("air data for london exists")
public void city_air_data_for_london() {
request = given().param("token", <token>).cookie("Cookie 1", "cookie one").filter(new ExtentRestAssuredFilter());
}
@When("retrieve the air data")
public void retrieve_the_city_air_data() {
response = request.when().get("https://api.waqi.info/feed/london");
}
@Then("the air data status code is {int}")
public void verify_status_code(int statusCode) {
response.then().statusCode(statusCode);
}
2. Test Execution
The tests can be executed with the ‘mvn clean install‘ command. The reports will be generated in the designated directory (reportDirectory reportDirectoryTimestamp).
It is advisable to place the allureResultsDirectory inside the project build directory so it can be deleted with the ‘clean‘ command. If the contents of the folder are not deleted then previous execution results will be displayed in the report.
3. Sample Report
Below is a part of the report generated from the execution of the above class. The request and response body contents are displayed as a browser pop up. The headers and cookies are displayed similarly as pop ups too, in a tabular fashion. These are available as links in the Spark report.
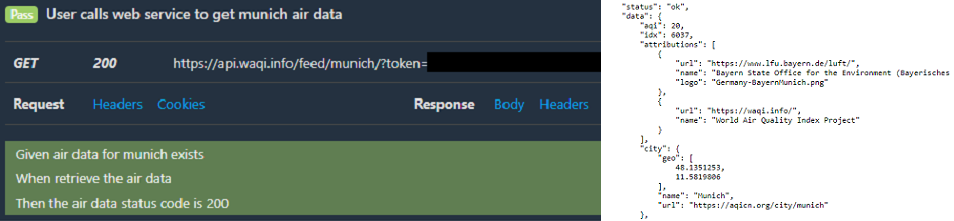
In the Pdf report, the details are available by clicking on the pin annotations. The details are shown in a browser pop up. The files are embedded inside the Pdf report and can also be viewed from View -> Navigation Panels -> Attachments.
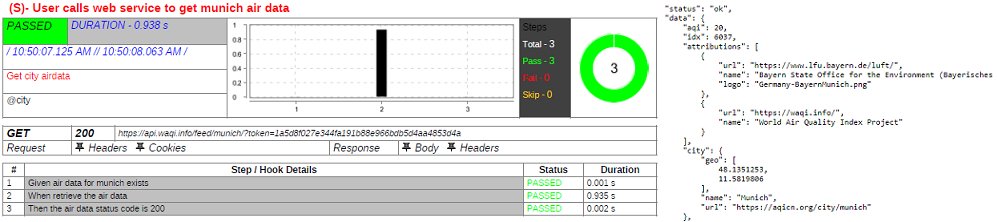
Report Additional Details
Following are some points which can be helpful in successful report generation.
1. Allure Results Directory
This is set by using the allureResultsDirectory configuration in the report plugin. The default value is ‘target/allure-results‘ directory path. This value also needs to be set as a ‘systemPropertyVariables‘ in the failsafe plugin. There are two points to consider.
First, if changing the value, remember to do it in both places, or better create a property and use it in both the locations.
Second, the contents of this folder need to be deleted before the test execution. Else the report will display results from previous runs. It is advisable to place the results directory in the project build so it gets deleted with the ‘clean‘ command.
2. Configuration File
The default path for the Spark report configuration is ‘src/test/resources/spark-config.xml‘. Place a file with the same name and location, will be picked up automatically. A custom file name and location can be set by using the ‘sparkConfigFilePath‘ configuration. If using a JSON file, this will need to be added.
The PDF report configurations can be modified by adding a yaml properties file named pdf-config.yaml, in the src/test/resources folder of the project. Refer here for more details.
3. System Info Properties File
The default path for this configuration is ‘src/test/resources/systeminfo.properties‘. Placing a file with the same name and location will be picked up automatically. A custom file name and location can be set by using the ‘systemInfoFilePath‘ configuration.
4. Blacklist Request and Response Headers
The header values can be hidden by using the ‘requestHeadersBlacklist’ and ‘responseHeadersBlacklist’ configuration. Multiple values can be added by using a comma delimited string as value. The value will be hidden by using the [BLACKLIST] text.
5. Spark Report View Order
The view order can be changed by using the ‘sparkViewOrder‘ configuration. The comma delimited values are from the ViewName enum. The default order is ‘TEST, CATEGORY, AUTHOR, DEVICE, EXCEPTION, DASHBOARD‘.
This doesn’t work at all. It generates no reports. I checked all comments and see that none of them were able to generate anything. I tried with the sample project as well.
Feel free not to use it. Thx.
hi, thanks for this awesome plugin , i’m facing following error when i add below mentioned json file in test/resource folder, if i json starts with object it’s working as expected and please guide is there any option to disable skipping [warnings] message
java.lang.IndexOutOfBoundsException: Index: 0, Size: 0
at java.util.ArrayList.rangeCheck(ArrayList.java:659)
at java.util.ArrayList.get(ArrayList.java:435)
at tech.grasshopper.processor.FeatureProcessor.updateScenarioWithBackgroundSteps(FeatureProcessor.java:28)
at tech.grasshopper.processor.FeatureProcessor.process(FeatureProcessor.java:23)
at tech.grasshopper.tests.ExtentTestHeirarchy.createFeatureExtentTest(ExtentTestHeirarchy.java:62)
at tech.grasshopper.tests.ExtentTestHeirarchy.lambda$createTestHeirarchy$1(ExtentTestHeirarchy.java:47)
at java.util.ArrayList.forEach(ArrayList.java:1259)
at tech.grasshopper.tests.ExtentTestHeirarchy.createTestHeirarchy(ExtentTestHeirarchy.java:45)
at tech.grasshopper.extent.reports.ReportCreator.generate(ReportCreator.java:28)
at tech.grasshopper.CucumberRestAssuredExtentReportPlugin.execute(CucumberRestAssuredExtentReportPlugin.java:123)
at org.apache.maven.plugin.DefaultBuildPluginManager.executeMojo(DefaultBuildPluginManager.java:137)
at org.apache.maven.lifecycle.internal.MojoExecutor.execute(MojoExecutor.java:210)
at org.apache.maven.lifecycle.internal.MojoExecutor.execute(MojoExecutor.java:156)
at org.apache.maven.lifecycle.internal.MojoExecutor.execute(MojoExecutor.java:148)
at org.apache.maven.lifecycle.internal.LifecycleModuleBuilder.buildProject(LifecycleModuleBuilder.java:117)
at org.apache.maven.lifecycle.internal.LifecycleModuleBuilder.buildProject(LifecycleModuleBuilder.java:81)
at org.apache.maven.lifecycle.internal.builder.singlethreaded.SingleThreadedBuilder.build(SingleThreadedBuilder.java:56)
at org.apache.maven.lifecycle.internal.LifecycleStarter.execute(LifecycleStarter.java:128)
at org.apache.maven.DefaultMaven.doExecute(DefaultMaven.java:305)
at org.apache.maven.DefaultMaven.doExecute(DefaultMaven.java:192)
at org.apache.maven.DefaultMaven.execute(DefaultMaven.java:105)
at org.apache.maven.cli.MavenCli.execute(MavenCli.java:957)
at org.apache.maven.cli.MavenCli.doMain(MavenCli.java:289)
at org.apache.maven.cli.MavenCli.main(MavenCli.java:193)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.lang.reflect.Method.invoke(Method.java:498)
at org.codehaus.plexus.classworlds.launcher.Launcher.launchEnhanced(Launcher.java:282)
at org.codehaus.plexus.classworlds.launcher.Launcher.launch(Launcher.java:225)
at org.codehaus.plexus.classworlds.launcher.Launcher.mainWithExitCode(Launcher.java:406)
at org.codehaus.plexus.classworlds.launcher.Launcher.main(Launcher.java:347)
[ERROR] STOPPING EXTENT REPORT GENERATION – Index: 0, Size: 0
“`json
[
{
“ticker”: “GMAB”,
“exchange”: “COSE”
},
{
“ticker”: “GMAB”,
“exchange”: “NASDAQ”
}
]
“`
Used refers project: “https://github.com/grasshopper7/cucumber-rest-assured-testng-report”
This will only work with the json report file generated by cucumber. What is this additional json you have added?
thanks for reply mounish, it’s test-data file for my project
try to keep the test data file outside the folder hierarchy that is configured for the plugin “cucumberReportsDirectory”
I downloaded the sample project – https://github.com/grasshopper7/cucumber-rest-assured-testng-report.git and ran both Runner Class and feature file, but can’t get the report generated.
I also created another project and imported all plugins and dependencies and also had no luck. Only the allure-results folder is being generated.
What am I doing wrong? Shall I enable anything else? At least to make the sample project work.
can you share your repository link?
https://github.com/grasshopper7/cucumber-rest-assured-testng-report.git
Hello Mounish, were you able to check the sample project on this problem?
Sorry I was really busy the last couple of days.
U shared my repo link. Can you share the repo with your code? Or you have made no changes to the sample code?
Are you getting any messages or exceptions in the console?
I appreciate you taking the time to respond, even though you are busy.
Yes, I cloned and have been checking out your sample project repository because it’s a good starting point for learning how to generate reports. I removed what I’d created to test because it was not working.
However, when I run RunCukeIT or any of the scenarios in book.feature or city.feature, I am not getting an HTML report generated. Only the allure-results folder is being generated. No code has been updated after cloning your repository.
No errors, except assertion errors created intentionally. I just can’t find any HTML report for the tests.
How can I Log info with extent adapter in BDD cucumber Rest assured
This code created new folder with allure-result, but still I am not getting the Rqst and response in html and spark report. Can you please help.
{
if (req == null) {
PrintStream log = new PrintStream(new FileOutputStream(“logging.txt”)); // For logging information in logging.txt file
req = new RequestSpecBuilder().setBaseUri(Getglobalvalues(“baseUrl”))
.addFilter(RequestLoggingFilter.logRequestTo(log))
.addFilter(ResponseLoggingFilter.logResponseTo(log)).setContentType(ContentType.JSON)
.addHeader(“Authorization”, “Basic ” + auth()).setUrlEncodingEnabled(true).addFilter(new ExtentRestAssuredFilter()).build();
// Requestspecbuilder will create the request and added Authentication
return req;
}
Sorry for the late reply. Have you tried with the sample project?
Hi team,
We are trying to configure Extent report for “Cucumber-Rest Assured” Project. We need to add the Request and Response info in the extent report. We are using Gradle as the build tool. Please let us know if this is possible. If so, please share the dependency details.
Hi, I have not tried it with Gradle. Give me a couple of days to figure it out. If you do not hear from me by Monday, drop me a comment. Thanks.
Hi Mounish, im trying too with gradle and i cant.
Hi Mounish, im trying too with gradle and i cant.
I have not tried with gradle. What is the error? Any console messages.
Any way to use it with mvn test instead of mvn install?
With mvn install any failed test prevents the reporter from generating a report. Adding “-Dmaven.test.failure.ignore” make it work localy, but the downside is that CI/CD is also ignoring fails and false positive results are displayed.
Well, actually with mvn test it would be the same, so it’s not the case. But anyway, having WITH failed tests would be great.
Hi,
I have run this multiple times on local computer with failing scenarios and the report is generated. Even ‘mvn verify’ works. I am not sure what I am missing.
Is there any exception related to the report code in the console? Any chance you can share a repo with the reproducible issue, for me to look at?
Thanks
Hi,
I’ve tried many different things, but no success.
I always get this error, when there is a failing test:
[ERROR] Failed to execute goal org.apache.maven.plugins:maven-surefire-plugin:3.0.0-M8:test (default-test) on project api-testing: There are test failures.
Try running the tests in the “integration-test” goal. U can run with mvn verify or mvn install
Hi,
I had someone complain about a similar issue with no report generated on failure with the PDF report. They provided no more info and I could not debug. Can you set the ‘pdfGenerate’ parameter to false and try?
Below are some issues I am facing:
* Extent Report is generated but only when Feature is passed. When any Test Scenario is failed, Report is not generated and no info about extent report is displayed. allure-results are generated.
* PDF report is not generated saying ‘PDF Configuration YAML’ file is not available, even though I saved it in src/test/resource folder
* Request and Response are not displayed in the Spark Report.
Below is the Info I got in Terminal:
[INFO] STARTING EXTENT REPORT GENERATION
[WARNING] Skipping json report at ‘target/cucumber-allure.json’, as unable to parse json report file to Feature pojo.
Dec 15, 2022 8:35:00 PM tech.grasshopper.pdf.PDFCucumberReport collectReportConfiguration
INFO: PDF report configuration YAML file not found. Using default settings.
Dec 15, 2022 8:35:02 PM tech.grasshopper.pdf.extent.RestAssuredExtentPDFCucumberReporter flush
SEVERE: An exception occurred
java.lang.NullPointerException
at tech.grasshopper.pdf.section.details.RestAssuredDisplay.display(RestAssuredDisplay.java:46)
at tech.grasshopper.pdf.section.details.RestAssuredDetailedSection.scenarioAdditionalInfoDisplay(RestAssuredDetailedSection.java:25)
at tech.grasshopper.pdf.section.details.DetailedSection.createSection(DetailedSection.java:56)
at tech.grasshopper.pdf.RestAssuredPdfCucumberReport.createDetailedSection(RestAssuredPdfCucumberReport.java:28)
at tech.grasshopper.pdf.PDFCucumberReport.createReport(PDFCucumberReport.java:96)
at tech.grasshopper.pdf.extent.RestAssuredExtentPDFCucumberReporter.flush(RestAssuredExtentPDFCucumberReporter.java:73)
at tech.grasshopper.pdf.extent.RestAssuredExtentPDFCucumberReporter.access$100(RestAssuredExtentPDFCucumberReporter.java:21)
at tech.grasshopper.pdf.extent.RestAssuredExtentPDFCucumberReporter$1.onNext(RestAssuredExtentPDFCucumberReporter.java:46)
at tech.grasshopper.pdf.extent.RestAssuredExtentPDFCucumberReporter$1.onNext(RestAssuredExtentPDFCucumberReporter.java:39)
at io.reactivex.rxjava3.subjects.PublishSubject$PublishDisposable.onNext(PublishSubject.java:310)
at io.reactivex.rxjava3.subjects.PublishSubject.onNext(PublishSubject.java:226)
at com.aventstack.extentreports.ReactiveSubject.onFlush(ReactiveSubject.java:83)
at com.aventstack.extentreports.AbstractProcessor.onFlush(AbstractProcessor.java:85)
at com.aventstack.extentreports.ExtentReports.flush(ExtentReports.java:279)
at tech.grasshopper.extent.reports.ReportCreator.generate(ReportCreator.java:30)
at tech.grasshopper.CucumberRestAssuredExtentReportPlugin.execute(CucumberRestAssuredExtentReportPlugin.java:112)
at org.apache.maven.plugin.DefaultBuildPluginManager.executeMojo(DefaultBuildPluginManager.java:137)
at org.apache.maven.lifecycle.internal.MojoExecutor.doExecute2(MojoExecutor.java:370)
at org.apache.maven.lifecycle.internal.MojoExecutor.doExecute(MojoExecutor.java:351)
at org.apache.maven.lifecycle.internal.MojoExecutor.execute(MojoExecutor.java:215)
at org.apache.maven.lifecycle.internal.MojoExecutor.execute(MojoExecutor.java:171)
at org.apache.maven.lifecycle.internal.MojoExecutor.execute(MojoExecutor.java:163)
at org.apache.maven.lifecycle.internal.LifecycleModuleBuilder.buildProject(LifecycleModuleBuilder.java:117)
at org.apache.maven.lifecycle.internal.LifecycleModuleBuilder.buildProject(LifecycleModuleBuilder.java:81)
at org.apache.maven.lifecycle.internal.builder.singlethreaded.SingleThreadedBuilder.build(SingleThreadedBuilder.java:56)
at org.apache.maven.lifecycle.internal.LifecycleStarter.execute(LifecycleStarter.java:128)
at org.apache.maven.DefaultMaven.doExecute(DefaultMaven.java:294)
at org.apache.maven.DefaultMaven.doExecute(DefaultMaven.java:192)
at org.apache.maven.DefaultMaven.execute(DefaultMaven.java:105)
at org.apache.maven.cli.MavenCli.execute(MavenCli.java:960)
at org.apache.maven.cli.MavenCli.doMain(MavenCli.java:293)
at org.apache.maven.cli.MavenCli.main(MavenCli.java:196)
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
at java.base/jdk.internal.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.base/java.lang.reflect.Method.invoke(Method.java:566)
at org.codehaus.plexus.classworlds.launcher.Launcher.launchEnhanced(Launcher.java:282)
at org.codehaus.plexus.classworlds.launcher.Launcher.launch(Launcher.java:225)
at org.codehaus.plexus.classworlds.launcher.Launcher.mainWithExitCode(Launcher.java:406)
at org.codehaus.plexus.classworlds.launcher.Launcher.main(Launcher.java:347)
[INFO] EXTENT REPORT SUCCESSFULLY GENERATED
Hi, Can you share a repo with the minimum code to reproduce this as I am unable to debug the error from the stack? I am able to generate the report successfully from the sample report code. Have you tried running the sample report? Thanks.
Hi Mounish ,
Hope you are doing good!!!
I want to add steps pass assertion log with user given message with expected and actual data in reporting. For example I have a below steps .
@Then(“^I verify the response code \”([^\”]*)\” in response$”)
public void verifyResponseCode(String responseCode) {
assertEquals(response.statusCode(), StatusCode.CODE_201.code);
assertEquals(response.as(Playlist.class).getName(), requestPlaylist.getName());
}
I want to know that if there is any assertion methods for printing the actual and expected data in the reporting steps.
Any suggestion and help would be highly appreciated.
Thanks
Anam
There is no built in method you can use which will combine assertion and logging as they are from two different frameworks, junit (or testng) and cucumber. But you can easily create a method which takes the actual, expected and scenario parameters. You can display the actual and expected values by using the scenario.log() method and also perform assertion in it.
Mounish, thank you very much for quick reply. I know about the Scenario.log method for printing the logs but I wanted to know if there is combined method for logging and assertion.
Now I will get the Scenrio object in the stepdefination file and just after performing the assertion will write the scenario.log for logging.
Thank you!!!
I’m trying to implement this to my project but if my Cucumber runner name isnt RunCukeIT the execution fails with a:
STARTING EXTENT REPORT GENERATION
tech.grasshopper.exception.CucumberRestAssuredExtentReportPluginException: No Cucumber Json Report found. Stopping report creation. Check the ‘extentreport.cucumberJsonReportDirectory’ plugin configuration.
STOPPING EXTENT REPORT GENERATION – No Cucumber Json Report found. Stopping report creation. Check the ‘extentreport.cucumberJsonReportDirectory’ plugin configuration.
Why? Why I cant name it CucumberRunnerTest or something like that?
You can name the runner any name you want as long as this name is included in the failsafe plugin configuration. Refer to this – https://maven.apache.org/surefire/maven-failsafe-plugin/examples/inclusion-exclusion.html
We have project with multiple TestRunner setup.
I have set value in all Runner classes in @CucumberOptions as shown below
plugin = {
“json:target/cucumber-json.json”,”tech.grasshopper.AllureCucumberMappingPlugin:target/cucumber-allure.json”,
The log always shows only 1 feature which is run in spite all the tests were run successfully. It is possible because the cucumber-json.json is overwritten every time new Runner class is run.
How do we solve this?
Yes, cucumber json file is getting overwritten. Please keep the json file names different in the runners. The plugin should be able to get the data from multiple cucumber-json files.
If not then it is a bug. Let me know.
Thanks
Hi Mounish,
I tried naming different for different json files. It doesn’t fix the issue. It always looks for cucumber-json file.
Can you please provide us a fix for this?
This is fixed in version 2.3.0. Should be available in maven central in a day or two. I will update the sample implementations and the article tomorrow. The mapping file paths need to be added as comma separated string to the ‘cucumberAllureMappingFiles’ parameter of the Maven plugin.
Documentation updated, refer to section ‘Cucumber Runner Configuration’ for configuration settings for multiple runners. Sample implementation committed
Thanks a lot, Mounish!
Hi, I’m following your example (https://github.com/grasshopper7/cucumber-rest-assured-testng-report) and I’m getting this error below. It seems that the error is related to the dependency Lombok version.
[ERROR] Failed to execute goal org.apache.maven.plugins:maven-compiler-plugin:3.1:testCompile (default-testCompile) on project spec-cukes: Fatal error compiling: java.lang.IllegalAccessError: class lombok.javac.apt.LombokProcessor (in unnamed module @0x94e51e8) cannot access class com.sun.tools.javac.processing.JavacProcessingEnvironment (in module jdk.compiler) because module jdk.compiler does not export com.sun.tools.javac.processing to unnamed module @0x94e51e8 -> [Help 1]
[ERROR]
[ERROR] To see the full stack trace of the errors, re-run Maven with the -e switch.
[ERROR] Re-run Maven using the -X switch to enable full debug logging.
[ERROR]
[ERROR] For more information about the errors and possible solutions, please read the following articles:
[ERROR] [Help 1] http://cwiki.apache.org/confluence/display/MAVEN/MojoExecutionException
WHat is the jvm version?
> java -version
openjdk version “18.0.2.1” 2022-08-18
OpenJDK Runtime Environment (build 18.0.2.1+1-1)
OpenJDK 64-Bit Server VM (build 18.0.2.1+1-1, mixed mode, sharing)
You can refer to this – https://stackoverflow.com/questions/66801256/java-lang-illegalaccesserror-class-lombok-javac-apt-lombokprocessor-cannot-acce
Maybe this can help.
I saw that same post. But I’m not using Lombok in my code. The error comes from your plugin that uses Lombok
The lombok dependency is coming from core extent report code on which I do not have any control. As far as I know, the extent report code has been tested upto Java 8. You could downgrade your java and try.
Actually the lombok dependency should have been included with ‘scope’ dependency. https://stackoverflow.com/questions/29385921/maven-scope-for-lombok-compile-vs-provided
It didn’t work. I’ve tried using java 8 and I’m having the same issue. BTW, I’m using this repository as is https://github.com/grasshopper7/cucumber-rest-assured-testng-report
Hello Mounish, I am getting following error
[ERROR] STOPPING EXTENT REPORT GENERATION – Unable to navigate Cucumber Json report folders. Stopping report creation. Check the ‘extentreport.cucumberJsonReportDirectory’ plugin configuration.
tech.grasshopper.exception.CucumberRestAssuredExtentReportPluginException: Unable to navigate Cucumber Json report folders. Stopping report creation. Check the ‘extentreport.cucumberJsonReportDirectory’ plugin
POM details
${allure.results.directory}
${project.name}/target
${project.name}/target/cucumber-allure.json
${extent.report.directory}
Cucumber Runner
plugin = { “json:target/cucumber-json.json”,”tech.grasshopper.AllureCucumberMappingPlugin:target/cucumber-allure.json” }
cucumber-allure.json and cucumber-json.json, both not generated. Please suggest.
Hi, Unfortunately the comments section strips out most of XML tags so unable to understand the configuration.
What is this configuration referring to – ${project.name}/target & ${project.name}/target/cucumber-allure.json?
Have you tried with the sample implementation POM? From your runner settings you can use the default settings for the cucumber json and cucumber allure json files.
The property name is not correct in the error message. It should be ‘extentreport.cucumberReportsDirectory’. Will look at this.
Hi Mounish,
Extent Reports fail to generate due to below error while executing via mvn clean verify or mvn clean install. Error Log below:
[WARNING] Skipping json report at ‘target\cucumber-allure.json’, as unable to parse json report file to Feature pojo.
tech.grasshopper.exception.CucumberRestAssuredExtentReportPluginException: No Allure Json Result found. Stopping report creation. Check the ‘extentreport.allureResultsDirectory’ plugin configuration.
at tech.grasshopper.results.AllureResultsCollector.retrievePaths(AllureResultsCollector.java:78)
at tech.grasshopper.results.AllureResultsCollector.retrieveResults(AllureResultsCollector.java:35)
at tech.grasshopper.CucumberRestAssuredExtentReportPlugin.execute(CucumberRestAssuredExtentReportPlugin.java:106)
at org.apache.maven.plugin.DefaultBuildPluginManager.executeMojo(DefaultBuildPluginManager.java:137)
at org.apache.maven.lifecycle.internal.MojoExecutor.execute(MojoExecutor.java:210)
at org.apache.maven.lifecycle.internal.MojoExecutor.execute(MojoExecutor.java:156)
at org.apache.maven.lifecycle.internal.MojoExecutor.execute(MojoExecutor.java:148)
at org.apache.maven.lifecycle.internal.LifecycleModuleBuilder.buildProject(LifecycleModuleBuilder.java:117)
at org.apache.maven.lifecycle.internal.LifecycleModuleBuilder.buildProject(LifecycleModuleBuilder.java:81)
at org.apache.maven.lifecycle.internal.builder.singlethreaded.SingleThreadedBuilder.build(SingleThreadedBuilder.java:56)
at org.apache.maven.lifecycle.internal.LifecycleStarter.execute(LifecycleStarter.java:128)
at org.apache.maven.DefaultMaven.doExecute(DefaultMaven.java:305)
at org.apache.maven.DefaultMaven.doExecute(DefaultMaven.java:192)
at org.apache.maven.DefaultMaven.execute(DefaultMaven.java:105)
at org.apache.maven.cli.MavenCli.execute(MavenCli.java:957)
at org.apache.maven.cli.MavenCli.doMain(MavenCli.java:289)
at org.apache.maven.cli.MavenCli.main(MavenCli.java:193)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:90)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:55)
at java.lang.reflect.Method.invoke(Method.java:508)
at org.codehaus.plexus.classworlds.launcher.Launcher.launchEnhanced(Launcher.java:282)
at org.codehaus.plexus.classworlds.launcher.Launcher.launch(Launcher.java:225)
at org.codehaus.plexus.classworlds.launcher.Launcher.mainWithExitCode(Launcher.java:406)
at org.codehaus.plexus.classworlds.launcher.Launcher.main(Launcher.java:347)
[ERROR] STOPPING EXTENT REPORT GENERATION – No Allure Json Result found. Stopping report creation. Check the ‘extentreport.allureResultsDirectory’ plugin configuration.
What is the value of ‘extentreport.allureResultsDirectory’ configuration you have in the POM? THe default value is ‘target/allure-results’.
Do you have files with the suffix ‘-result.json’ in this directory?
Thanks
this is the path for extentreport.allureResultsDirectory
${project.build.directory}/allure-results
report
after tests are run a folder named ‘allure-results’ with various files with suffix ‘-result.json’ is also generated. Only thing its placed inside sub modules folder rather than inside it’s target folder. also under sub-module report folder with timestamp is also created but is blank with only a folder data inside.
Set the extentreport.allureResultsDirectory value to a path which takes into account the sub module part. Let me know if this works. Thanks.
${project.build.directory}/allure-results
${project.build.directory} this evaluate to target folder inside current sub-module and I tried changing to other paths but nothing works
Let me try it out. U have a maven module inside the parent project and you are executing the parent POM. Is that the setup you have?
Created a repo – https://github.com/grasshopper7/ra-cuke-parent
Check the child module POM – https://github.com/grasshopper7/ra-cuke-parent/blob/master/ra-cuke-child/pom.xml.
U JUST need to add two configurations – cucumberReportsDirectory & cucumberAllureMappingFile in the plugin. Clearly marked with comment – “START EXTRA CONFIG”.
No need to change the “allureResultsDirectory” config.
THen execute the parent POM.
Hi i am used this pom,
but still getting the above mentioned error.any help
Hi, Can you add some more details? Is it a multi module project? Is the example repo not working?
Hi ,
I implemented this reporter but
The html file can’t shown the any attachments even allure data file and cucumber-allure.json are created, I check your example
project, When the report is created, I can see only on different that cucumber-allure.json created as following on my project
example project allure.json :
{
“classpath:stepdefs/book.feature:31” : “10fc4316-6dd7-4453-b294-e3d25e64002e”,
}
My project allure.json :
{
“file:///Users/yahya.kara/Documents/projects/ScApiTesting/src/test/resources/features/Login.feature:8” : “3d8e8a13-ae52-42af-b331-ce53c10e7b43”,
}
Could you help me about this
I found a bug in the parsing logic. Currently the code is working when the runner uses the default step definition locations. Will fix and upload new version.
This has been fixed now. U will need to upgrade the plugin version to 2.1.2. And other jar versions need to be upgraded also. Check the ‘POM Configurations’ section of the article. Let me know if you have any issues.
Took longer then expected as Maven was replicating the jars pretty slowly over the past week. Thanks.
This maven dependencies are missing
https://github.com/grasshopper7/cucumber-rest-assured-testng-report/tree/cdacf4d84ab1d78974d9a58bd9a463ca440f3afe
[INFO] ————————————————————————
[ERROR] Plugin tech.grasshopper:cucumber-rest-assured-extent-report-plugin:1.1.1 or one of its dependencies could not be resolved: Failure to find tech.grasshopper:cucumber-rest-assured-extent-report-plugin:jar:1.1.1 in https://repo.maven.apache.org/maven2 was cached in the local repository, resolution will not be reattempted until the update interval of central has elapsed or updates are forced -> [Help 1]
[ERROR]
The dependency is available at https://mvnrepository.com/artifact/tech.grasshopper/cucumber-rest-assured-extent-report-plugin/1.1.1. Also its dependencies are available.
I tried running this and it worked, after deleting all my local jars and downloading fresh from maven.